How to fix the Symfony web profiler in Apache
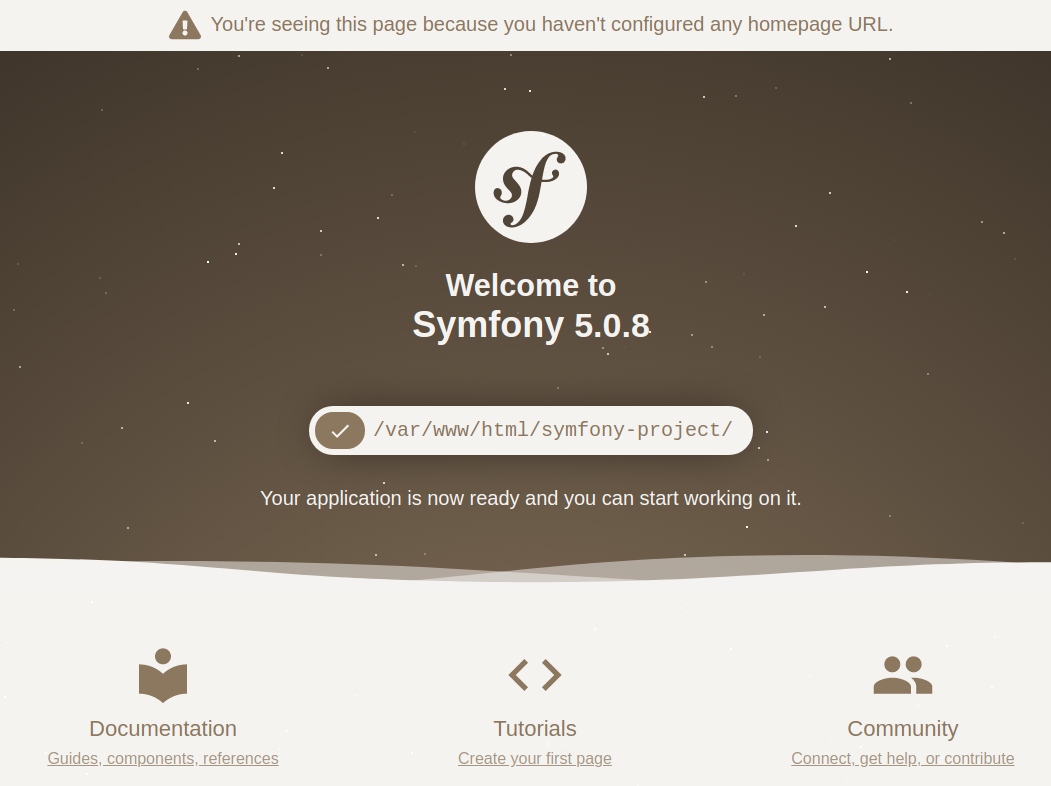
So you've decided you want to create a web project using Symfony 5. Great! By now you've got the Welcome to Symfony 5.x.y message on screen; great. You're going to need the Apache pack to ensure the custom routes work nicely with the Apache HTTP Server. Run the following at the root of the project to download the pack, and have it create the .htaccess file for the project:
composer require symfony/apache-pack
If you're going to be building something, you're going to run in to errors and problems; you're going to want to debug. Fortunately there's the Symfony profiler component to help you. Again, at the root of the project, run:
composer require --dev symfony/profiler-pack
Reload the page you're on, and you should be presented with the welcome screen, shortly followed by:

If, however, you're getting the following error:

This simply means the rewriting of URLs isn't working within Apache. There's two things to check:
- mod_rewrite is enabled
- Apache is set to AllowOverrides
Enable mod_rewrite
If you've been able to get this far, there's a good chance you've set up the virtual host for Apache, and enabled it. You may not have enabled the rewrite module. To do this, open a terminal window, and run the following (this assumes you are running Ubuntu, or other Debian based Linux distribution):
sudo a2enmod mod_rewrite
sudo service apache2 restart
Try loading the page again. If all has worked, great! You are ready to develop your application, and use the profile toolbar to help you diagnose issues.
Allow Overrides in Apache
This change may need to be made in two places, but is the same in both. Firstly, in the file /etc/apache2/apache.conf locate the section with the following text:
<Directory /var/www/>
Options Indexes FollowSymLinks
AllowOverride None
Require all granted
</Directory>
Change the AllowOverride line from None to All:
<Directory /var/www/>
Options Indexes FollowSymLinks
AllowOverride All
Require all granted
</Directory>
Save the changes, and open your virtual host configuration in /etc/apache2/sites-available
If there's no <Directory>
directive, one will need creating. Using the example above, add the following section (or modify the existing one) to:
<Directory /var/www/symfony-project/public/>
Options Indexes FollowSymLinks
AllowOverride All
Require all granted
</Directory>
Save the changes, and restart Apache. Once you reload the page, the profile toolbar will show as being a 404 error. This is expected, as Symfony can't find anything to route the request to.
Want to see it with a 200 response?
Open the file config/routes.yaml in your Symfony project, and uncomment all 3 lines so it looks as follows:
index:
path: /
controller: App\Controller\DefaultController::index
Then, in the project folder src/Controller, create the file DefaultController.php. Set the full contents of the file to be:
<?php
namespace App\Controller;
use Symfony\Bundle\FrameworkBundle\Controller\AbstractController;
use Symfony\Component\HttpFoundation\Request;
use Symfony\Component\HttpFoundation\Response;
class DefaultController extends AbstractController
{
public function index(Request $request): Response
{
return new Response("<html><body><p>Hello World</p></body></html>");
}
}
Save it, and refresh your project page. The following should be displayed:

There you have it, the Symfony profiler is installed and working for both error responses, and success responses.